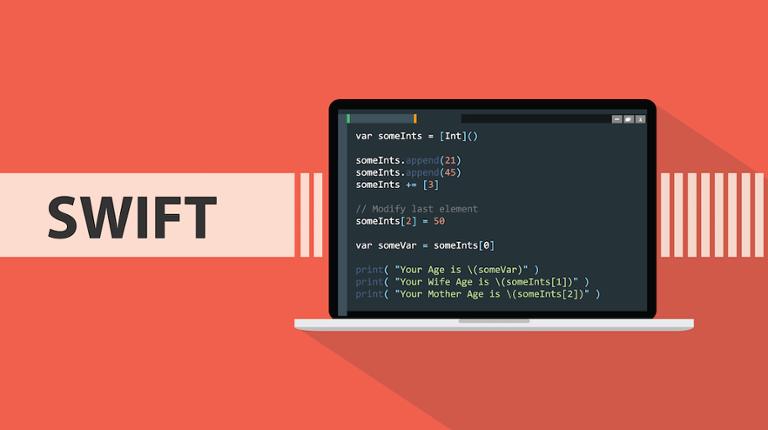
Within the Swift programming language, loops play an important role. For anyone just learning the language (in which case, we can humbly recommend our other articles on arrays, strings, sets, and more), Swift loops can help make large, cumbersome tasks simple.
In this article, we’ll walk you through loops: What they are, why you’d want to use them, and the major types of Swift loops you’ll want to master.
What are Swift Loops, and Why Do You Use Them?
Loops are part of control flow, which is a clever way of saying they help you accomplish a task several times. It helps you iterate over data collection types like arrays and dictionaries, so you can do things like greet people, or return values.
There are two core types of loops: for-in loops, and while loops. A for-in loop allows you to iterate over a sequence of values, such as an array or characters in a string. While loops perform a task under Boolean conditions; it will do a thing so long as something else is (or isn’t) happening.
If you’re a bit lost, don’t worry—coding isn’t always easy! We’re about to get into examples that should hopefully clear things right up.
Using a For-In Loop
Let’s do the ol’ copy-paste from an example we used in our Swift Arrays piece:
let names = [“Nate”, “Nick”, “Neil”]
You could use a for-in loop to greet each person, like so:
let names = [“Nate”, “Nick”, “Neil”] for name in names { print(“Hello, \(name)!”) }
Okay, so let’s dissect this one just a bit. First, we have our array, named “names.” That’s just storing names of people. The loop is then calling the array and iterating through it one-by-one.
The for-in loop is essentially telling your compiler: "For every name in the ‘names’ array, I want to print a greeting, and that greeting is in my print statement."
You can also iterate over a dictionary with key-value pairs. Let’s say I wanted the greeting to add a bit more context to whomever was being greeted:
let namesAndLocations = [“Nate”: “Oregon”, “Nick”: “New York”, “Neil”: “Colorado”] for (name, location) in namesAndLocations { print("Hey, \(name) from \(location) is here!") }
Dictionaries are based on key-value pairs (like “Nate”: “Oregon”), but the compiler infers their type so you don’t have to express it in code. You could also make the location a seat number, and the greeting could tell them where they’re seated.
For-in loops are great for those times you want to go through an array or dictionary step-by-step—but what if you don’t?
Swift 'While' Loops
One key thing to remember about while loops: They rely on Boolean logic, meaning something is true or false, no in-between. This will drive your decision-making process for when to use a while loop.
Let’s jump right in with an example:
var i = 1 while i <= 10 { print(i) i = i + 1 }
This while loop simply understands the value of “i” is 1, and while the value of “i” is less than or equal to 10, it will print its value. Then it will add 1 to “i” and start the loop over again until the value of “i” is 10. Then the loop stops.
It stops printing the value of “i” at 10 because, in this case, 11 would be out of range (or false). We explicitly told it to stop when “i” was equal to ten. You can manipulate the values as you like (make “i” equal zero, turn 10 into 100, and add 5 each time the loop completes, for example) and the logic remains the same (in that example, it will add five each time it goes through the loop until it hits 100, then stop).
Repeat-While Loops
There is also the repeat-while loop, which is used when you have to make at least one pass through your code and return a value. This is often used in gaming, where a player needs to do something like roll dice. It’s much less cumbersome to code a repeat-while loop for rolling dice than it would be to hard-code all sorts of variables into a while loop.
Here’s an example:
let finalSquare = 25 var board = [Int](repeating: 0, count: finalSquare + 1) board[03] = +08; board[06] = +11; board[09] = +09; board[10] = +02 board[14] = -10; board[19] = -11; board[22] = -02; board[24] = -08 var square = 0 var diceRoll = 0 repeat { // move up or down for a snake or ladder square += board[square] // roll the dice diceRoll += 1 if diceRoll == 7 { diceRoll = 1 } // move by the rolled amount square += diceRoll } while square < finalSquare print("Game over!")
This is a great example we pinched from the Swift Programming Language documentation. It has a board with 25 squares, and is modeled after the “snakes and ladders” (or “chutes and ladders,” if you prefer) board game. In the first bit of code, you see snippets such as board[03] = +08
, which tells us that on the third square of the board, a ladder moves you eight positions to ’11.’ A negative value means there’s a snake (or chute) to slide you backwards.
The repeat-while loop allows you to roll the dice and move forward the number of places represented on your dice roll. It repeats this while you’re still on the board. Once you’ve successfully moved past the 25th position on the board, it prints: “Game over!”
Conclusion
Loops are one of the handiest tools you’ll use in Swift. They’re simple enough to grasp quickly, but complex enough that you’ll find yourself using them often in some pretty weird scenarios.
Also remember: Loops are logical expressions for code, so think about why you may want to use them before you start coding.