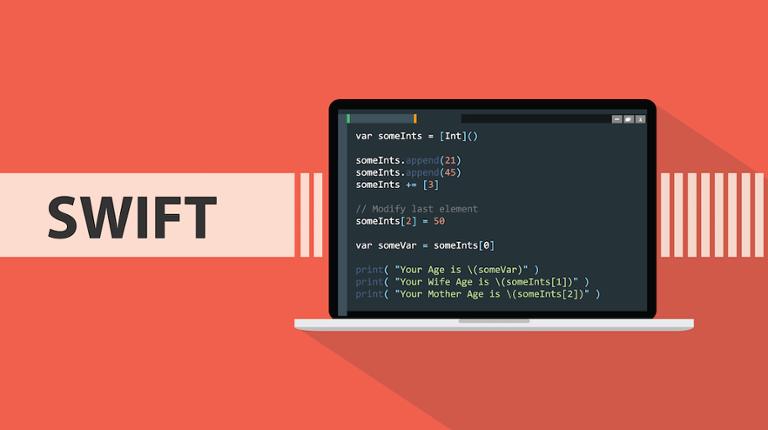
Swift enums aren’t quite as easy to master as some other aspects of the Swift programming language, but it’s a feature you should familiarize yourself with early in your quest to learn Swift. Though enums (enumerations) are found in other languages, Swift’s use of it is likely more flexible than you’ve come to experience in other languages.
Enums in Swift can be strings, characters, or any integer/floating-point. When you introduce an enum in your code, it is often named using uppercase syntax. This isn’t mandatory, but some prefer it… and Apple recommends it. Other types, such as properties or methods, use lower-snake-case syntax. We’re relying on the more familiar lower-snake-case syntax here.
(If you’re just joining us, we have a variety of other Swift micro-tutorials: check out how to work with functions, loops, strings, sets, arrays, and the Swift Package Manager.)
enum Seasons
Here, we’ve declared the enum ‘Seasons.’ Again, note how it’s capitalized. If we’d declared a constant, it would probably look something like this:
let annualSeasons
So Why Use Swift Enums?
The examples above are a good starting point on our journey into enums. If we’d used the constant to declare seasons, we could declare it thusly:
let annualSeasons = “Spring, Summer, Fall, Winter”
While this is accurate, it doesn’t let us do much. This is just a list of the seasons; with enums, we’re declaring the seasons and allowing them to be used elsewhere.
Enums are a bit like functions insomuch as they allow you to bundle some logic inside brackets. To get similar results with an enum, we’d do this:
enum seasons {
case spring
case summer
case fall
case winter
}
(Note the ‘case’ declarations do not have to be capitalized, but you can if you like. Just remember: The code is case-sensitive. If you were to call ‘Fall’ later in this code, it wouldn’t reference anything; but ‘fall’ will work.)
Here, we’ve declared the enum ‘Seasons,’ and given each season a case. In doing so, we’re allowing the use of said seasons later on in our code. The enum houses the logic and reference points in a reusable package.
How to Use Swift Enums
Let’s iterate on the ‘Seasons’ enum with dot syntax and a Switch case. After we declare ‘Seasons’ with all its cases, we declare a variable, and reference our enum:
var seasonNow = seasons.fall
Declaring a variable allows you to call that variable and get a value, because enums are value types in Swift. All we’re doing is saying: ‘This is the season now, and it’s Fall.’
As-is, this doesn’t do much on its own. A switch case will help. A switch allows you to iterate through your enum, and provide something in return. Here, we’ll use a simple print statement to tell the user how to dress for the season they’re in:
switch seasonNow {
case .spring:
print("It's cool out, wear a tee shirt!")
case .summer:
print("It's so hot! Time for a swim.")
case .fall:
print("Getting chilly. Bring a sweater!")
case .winter:
print("Bundle up, it's cold out”)
}
Let’s unpack this one just a bit; it’s probably a bit different than what you’re used to.
First, the switch case uses the name you declared for your variable. The enum holds the logic, and your variable allows you to access the logic. Using the same name in your switch case allows you to access the variable.
Think of it like a locked room. The enum is the room itself, full of all kinds of cool stuff. The variable is the locked door. Your switch case is the key to that door; it allows you to access what’s inside, and come and go as you please!
After we call the variable in our switch, we access the same cases as our enum declared. Here, we use dot-syntax, which is like your code saying: ‘Okay, full stop, it has to be (x), right?’
The dot-syntax is absolute. The colon after the case is declaring exactly what it will return. Here, your code is essentially telling you that if the season is absolutely Spring, and you’re sure, it’ll tell the user to wear a tee shirt.
Remember when we called seasons.fall
on our seasonsNow
variable? In doing that, we’re telling our code it’s Fall, and then telling the print statement. In Xcode, if you were to enter seasonNow = seasons.winter
, it would print "Bundle up, it's cold out” instead.
One Last Thing…
Let’s run through one final exercise on our enums basics. Let’s say you lived in a climate where Fall, Winter, and Spring were all cold… but Summer was warm. In this case, you’d always bundle up unless it was Summer. Your code could look something like this:
enum seasons {
case spring, summer, fall, winter
}
var seasonNow = seasons.summer
switch seasonNow {
case .summer:
print ("It's so hot! Time for a swim.”)
default:
print (“Bundle up and wear layers.”)
}
Multiple cases can appear on a single line. This is most useful when you have a ‘default’ statement in your switch case, and only need to declare something specific for a few cases (or ‘iterating’ over an enum). If we had 20 seasons, but only cared to dress light in four of them, this would be ideal.
Conclusion
Enums are pretty powerful. We’ve covered the basics, but you can do a lot with Swift enums and switch statements. Cases within switch statements can even house logic, which can be returned in a print statement.
For now, we encourage you to tinker with enums on your own in an Xcode playground.