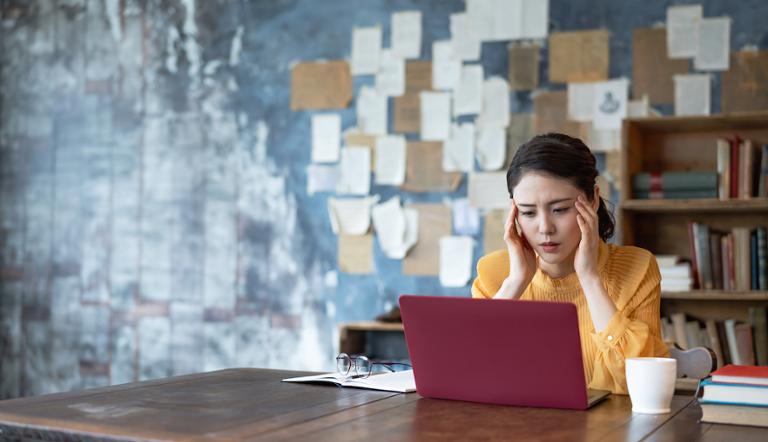
If you’re a C# developer interested in learning how to write AI apps, there are some libraries you’ll want to learn, along with some cloud services. Here’s how to get started without having to switch from C# to the most popular AI language, Python.
AI Concepts
First you’ll need to make sure you understand the concepts, including:
- Generative AI: This is today’s most popular AI, where the AI tools draw on what they’ve learned and generate new content. (The “answers” that ChatGPT gives you are an example, as are the generated AI images.)
- LLMs and other models: These are the data structures where the information lives. They’re usually “trained,” whereby they’re fed huge amounts of information.
- Machine Learning (ML): This is the branch of AI where systems learn patterns that they can use to make predictions.
- Natural Language Processing: This is a field of AI that allows software to understand and generate human language such as English.
These topics extend beyond just C#, which means you can study them in the context of other languages if necessary, such as Python or JavaScript. While learning these topics you can dive right in exploring the libraries and AI services we discuss next.
Cloud API Options
Because Microsoft built both Azure and the C# language, they have recently started adding .NET libraries that work directly with the AI services available on Azure. Azure has a wide array of AI tools, including APIs for OpenAI. Although OpenAI is a separate company from Microsoft, the latter’s massive investment in the former ensures they work closely together. Azure includes a set of OpenAI APIs that let you access the same models used by ChatGPT.
One such new C# library is called Microsoft.Extensions.AI. At the time of this writing, the library is still in early preview release, but you can start using the early features. This library is part of a bigger .NET AI initiative from Microsoft, which you can learn about here. Within that page are practice apps to help you get started, including creating a chat app, generating content, and summarizing text.
Remember, generative AI is all about generating content and producing natural human language. The “summarizing text” sample shows you how to send text to a large language model, and have it produce a summary. You can find the instructions here.
There isn’t much code in the example, but take the time to understand each line, even the first lines that import the libraries. Also notice that the calls to the API are asynchronous. Make sure you understand how that works, as well.
Another page on Microsoft’s site that you’ll find helpful is the AI for .NET developers home page. There are many more examples there to explore.
Note that all these samples can be run locally on your own machine, as they connect to the OpenAI APIs where the actual AI work is done. (If you’re looking at ways to do AI locally on your own GPU, we cover those later in this article.) At the heart of all this is a library called the Semantic Kernel, which manages the “back and forth” between your app and the Azure OpenAI APIs. Take a look at its main page here; spend some time going through the documentation and trying out the samples.
For example, on the left of the page is a section called Embedding Generation. Embeddings are an important part of today’s generative AI. Although you can build AI-powered apps without understanding them, knowing how they work will help you architect your apps better. Embeddings deal with converting sentences into tokens (that is, numbers for each word or part of a word) and then arranging these tokens into a vector. Those vectors can then be compared by “closeness” to the vectors for other sentences. The example in this section shows how you can create a couple sentences and send them to the Azure OpenAI APIs to be transformed into vectors.
Another important part of today’s AI is “Retrieval-Augmented Generation” (RAG) which is really a fancy term for modern text searches. With this technology, you combine your own documents and an LLM, allowing for complex searches that go beyond simple exact-word matches. For example, if your documents are about animals, and one entry about tigers explores sleeping habits, a user could use your app to search for words that are similar but not actually found in the document, such as “Tell me about nocturnal felines,” and the app would locate the article about tigers. (The Semantic Kernel has an example for doing such text searches, which you can find here.)
We’re only brushing the surface of what’s in this entire .NET AI initiative, but the above links are a great place to start.
Libraries and Frameworks
As with many Microsoft tools, what we described above is a great way to add AI to your C# apps; however, you’re getting a bit of vendor lock-in. What if you want to deploy a server on AWS, install your own LLMs, and roll your own AI apps? There are libraries that can do that for you.
Before you proceed, here are topics to explore:
- Models: Become familiar with the different types of models and when and where you use them. We can’t list them all here, but ask ChatGPT to describe the different types of models used within Generative AI. Look up terms like Embedding Models, Large Language Models, Text-to-Speech Models, Speech-to-Text Models, and Transformer Models.
- Hugging Face: Spend some time on the Hugging Face website, where you’ll find a wealth of tools for AI developers, including free models and libraries. (Presently there are over a million models available.)
- GPUs: Understand the hardware requirements. AI apps can technically run on a regular computer’s CPU, but it’ll be a very slow process. Instead, you need a GPU, typically one made by Nvidia that supports a technology called CUDA. This allows you to run code on the the thousands of cores on the GPU.
- Cloud compute: Understand how to launch servers on a cloud platform such as AWS. You’ll probably also want to learn how to work with containers such as docker, as these can run on cloud platforms as well.
It’s critical to learn about pricing on the cloud platform you’re using. If you launch a GPU instance on AWS, you’ll want to start it up, run your app, and turn it off as soon as the app is finished—otherwise you might get hit with a bill in the range of several hundreds of dollars, given the processing requirements.
Now on to the libraries. There are several open-source and free libraries available to C# developers to be able to work with AI models such as LLMs. Most of these libraries were originally built for Python developers but have since been ported to other languages. Here are the important ones; we’re presenting these in the order that we recommend you learn them in.
TensorFlow.NET: This is a .NET binding to a framework called TensorFlow, which is currently the most popular AI library. TensorFlow was created by Google. It’s technically a machine-learning library, but it transcends much of today’s AI. For example, it includes natural language processing tools, and generative AI. Note that this C# version of TensorFlow is not actually built by Google; it’s a community built tool that provides a .NET wrapper around TensorFlow.
Pro Tip: The community that built TensorFlow.NET is called SciSharp and they have other tools that you can explore. If you’re interested in data science and have heard of the cool tools available for Python, this group created several C# ports, including Numpy.NET (a port of NumPy) and Pandas.NET (a port of Pandas). You can see them all here.
ML.NET: This is a library created by Microsoft for machine learning. This library lets you do tasks such as train a machine learning model or use pre-trained models. It also includes data science tools that compete with some of the Python offerings such as NumPy and Pandas.
ONNX (Open Neural Network Exchange): This is both an organization and a library. The goal of the organization is to standardize models so that they can be used interchangeably between different tools and libraries such as those we’re describing here. The library implements this purpose. There is a C# binding for the ONNX library available here. These packages are available through the NuGet .NET package manager.
PyTorch for .NET: PyTorch is a python library with features similar to TensorFlow. There are a couple of open-source bindings available so you can use it from C#. These are called TorchSharp, which is built by the open-source .NET team, and Torch.NET, built by the same SciSharp people who built TensorFlow.NET. PyTorch is an older library, with roots as far back as 2002. It’s been continually developed and worked on since then, integrating modern AI features. However, it’s a little more difficult to learn compared to TensorFlow.
Conclusion
While Microsoft is creating a large AI ecosystem for both Azure and C#, there aren’t as many open-source and third-party libraries for executing AI in C#. When learning AI in the context of C#, plan to become very familiar with the offerings from Microsoft that we discussed in the first half of this article, as well as the one library called ML.NET.
It’s also important to become familiar with the other libraries we’ve mentioned, as that’s where much of the groundbreaking AI research is happening. And consider adding Python to your AI toolbox, given the language’s importance to the evolution of the AI ecosystem.