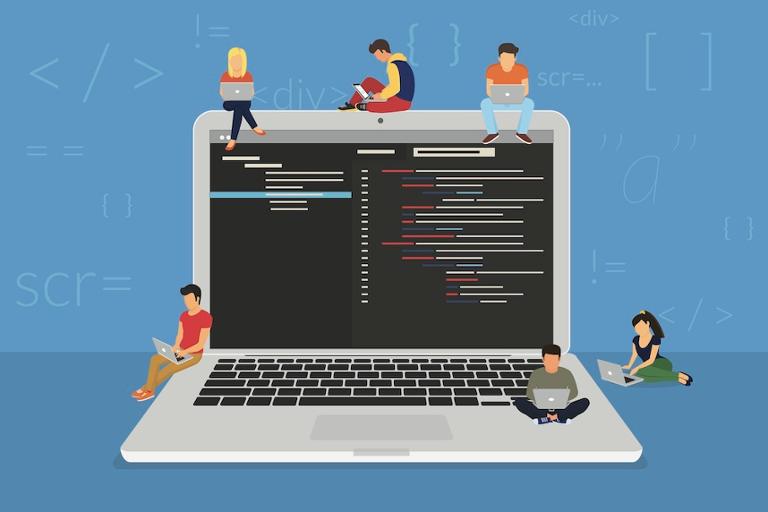
Although Git is integrated in many different editors, such as Visual Studio Code, it’s important to also know how to use Git from the command line. If you’re new to Git and spend some time in the official documentation, you might find it overwhelming. However, the reality is that the majority of your work in Git will likely only require a handful of commands. Once you have these commands down, you can then explore more advanced concepts for the rare occasions when you need to step outside of the norm.
The most common commands for Git involve saving your files and changes to the staging area, and committing those changes to source control, as well as viewing a list of files that have changed. Another common issue is deleting files. Let’s look at these.
Adding Changes
Remember from our last installment that your repository includes a staging area where you gather everything up that you want to commit to source control as a single batch. The staging area can include new files that you are adding to source control, as well as changes to your existing files, and file deletions. As you saw last time, to add files to your repo, you first add them to the staging using the Git add command:
git add one.js two.js
This example adds two files to the staging area (one.js
and two.js
). When you’re editing files, the simple process of changing those files does not store the changes in source control. You have to explicitly tell Git that you’re going to commit those changes by similarly adding them to the staging area.
First, I’m going to show you the long way to do it; then I’ll show you a shortcut. The long way is to again use the add command, even though the file is already in source control:
git add three.js
This might seem counterintuitive, but in fact we’re putting the modified three.js
file into the staging area; think of it as adding another change to the staging area.
Between the above commands, we now have two new files and one changed file all in the staging area. We can commit these files to source control:
git commit -m 'My changes'
This commits our files and includes a description (a “message” hence the -m
option) along with it (Git will not allow you to commit without adding a message). This is all fine, but if you’re only making changes to files that are already in source control, you can tackle the steps of adding to staging and committing to source control in a single step, like so:
git commit -a -m ‘My Changes’
We added the -a
option, which is a shortcut. This will take any files that are already in source control, place them in staging, and then commit those staged changes into source control, all in a single step. For most of your day, this is how you’ll likely do it. You already have all your files added to source control; you’ll spend time editing several files; then, when you’re ready to commit those changed files to source control, you type the commit command -a
and -m
options. No add is needed.
When wouldn’t you use this shortcut? On rare occasion you might be modifying multiple files but only want to commit a subset of those files. The commit command with -a
would stage and commit all the changed files, provided they’ve been added to source control. If you want to only commit some of them, you can add those changed files manually to staging first, and then commit without the -a
option.
Keeping Track
How do you keep track of what is (and isn’t) in staging, and what has (and hasn’t) been added to source control? Git provides a command called status:
git status
The output from this command includes three sections: The names of files you’ve added to staging (if any); the names of files already in source control that you’ve modified; and the names of files and directories that haven’t been added to source control. Here’s an example output:
On branch master
Changes to be committed:
new file: three.js
new file: four.js
Changes not staged for commit:
modified: one.js
Untracked files:
five.js
misc/
six.js
This tells me I have two new files, three.js
and four.js
, that I’ve placed in staging to be added to source control. It then tells me I have one file already in source control, called one.js
, that I’ve changed but have not added to staging. Finally, it tells me I have two files, five.js
and six.js
, and an entire directory called misc
that I have not added to source control. Those two files and one directory are effectively ignored by Git. (Quick quiz: If I do a commit right now without the -a
option, what will get committed? Answer in the conclusion.)
If you want to add five.js
and six.js
to source control, you can simply use the add command. But what about the files under misc
? If you want to add everything inside misc
, including any subdirectories and files in those subdirectories, you can add it all at once with a single command:
git add misc
That’s a lot easier than manually adding them one by one!
Deleting Files
Finally, let’s look at deleting files. There are two ways you can delete files; using the operating system itself like so:
rm myfile1.js
Or you can tell Git to delete the file:
git rm myfile2.js
The first approach, using the regular rm
command outside of Git, will be viewed by Git as a change to the file. It will show up in the second section of Git status. You’ll still need to add the change to the staging area (via git add myfile1.js
) and then commit the change. The second approach, using Git rm
, will do the same but in a single step. It will delete the file and then add the change to the staging area. Then, when you’re ready to commit the change, you run Git commit as usual, with the -m
flag and a message.
Conclusion
As you work with Git, the status command will be your daily friend. It will help you see exactly what files you’ve changed, what new files you need to add to source control, and what changes are sitting in the staging area. One piece of advice I have is to avoid keeping files in your directory that you have no plans to add to source control. That will keep your status output clean.
Oh, and the answer to the quiz question: If I were to do a commit without the -a
option, Git will only commit the files that are presently in staging. That means it will add three.js
and four.js
to source control. Because I have put my changed one.js
file into staging, that fill will not get committed.