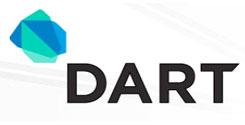
In my first tutorial about working with Dart, we looked at how to use its editor, definitely the best way to learn your way around. Now, we'll get ready to do some real work.
First, A Tip
In Tools, Preferences you'll find an Update tab. This lets you specify whether you want to manually run the Editor, or have it updated automatically. Once you download the editor, go to Help, About and click Apply, Update. The editor's updated about once a week.
In this tutorial, we'll look at some variable types and examples of their use. Bear in mind that much of what you're familiar with in JavaScript is also present in Dart. But it has objects with classes, as opposed to the prototype style of objects. The variable typing is stronger as well, which is meant to prevent errors occurring at run-time. To run shorter examples, create a new application in the Dart editor and wrap the examples inside this skeletal program. These are all short, so I've just listed them. In the future, longer applications will be attached as zip files of their source code. void main() { ... }
Numbers in Dart
There are two types of numbers in Dart: ints and doubles. You declare instances of them using the keywords var, int, num or double. Use var if the variable can be dynamic and hold any type of variable. That's more or less as it is in JavaScript. Use int or double to explicitly declare the type of the variables. The compiler will complain if you assign the wrong type in that case. This works: var a=0; a ="My name is David"; print(a);
But the snippet below generates an error because the compiler identifies b as an int that is wrongly being assigned a double value. This is type-checking in action, and one of the many ways that Dart improves on JavaScript. int b=7898765; b = 9.8; // ERROR Can't assign double to int
But we can use num instead of int. num b=7898765; b = 9.8; // This works
The int and double types are inherited from the type num. So anything declared as num can be used as either an int or a double at any time during the life of that variable.
Const and Final
These can be used as a prefix to var, num etc., which means that the value is fixed and can't be changed. But they are subtlety different. You can think of them as providing a way to make a variable read-only after the variable is initialized. For example: final a; // compile error
is an error. final String a="David"; final count =9;
I believe if you don't have an explicit type specified, then it's as if var is used. For scalar -- that is single -- variables, const is like final. You could replace final with const in the above example. However const can't access anything at run-time, it has to be fully evaluated when the program is compiled. Here's a small program that won't compile: import 'dart:math';
void main() { final count2 = sqrt(PI); const count3 = sqrt(PI); // this line won't compile print (count2); } The import line provides access to the dart:math library functions. See documentation below. I'll cover import in greater depth in the future, so save your questions. The constant value PI comes from the dart:math library as does the function sqrt() for calculating the square root. But the sqrt() function can only be called when this program runs and not at compile time so the const line is flagged as an error. The const keyword also applies to objects and collections, so we'll return to that in a future tutorial.
Bool Type
The boolean nonzero values that are the equivalent of true in JavaScript can be the cause of much difficulty, thanks to the implicit boolean conversions. To see how confusing this can be, read this post by Ben Nadel. If you get those wrong, it wastes a lot of time debugging. Anything that is a null object is false. Dart has an explicit bool type that takes just true and false. That means expressions like if (1) no longer work. void main() { if (1) { // won't compile print("true"); } }
JavaScript developers have to start thinking more like C# developers than C developers when they switch to Dart! By limiting conditional expressions to only checking against bools in this way, it makes for a lot less troublesome code. Here's an example of using a bool with other conditions. It prints false. void main() { int value=1; var CanPrint=false; if ((value & 1)== 1 && CanPrint) { print("true"); } else; { print("false"); } }
In my next tutorial I'll look at Strings and Container classes, such as Lists and Maps. As a last thought, you'll find the O'Reilly book Dart: Up and Running to be a useful online resource. Located on the Dartlang.org website, it's licensed under Creative Commons.
Related Links
- Dart: Up and Running [Dartlang.org]
- Dart math library documentation [Dartlang.org]