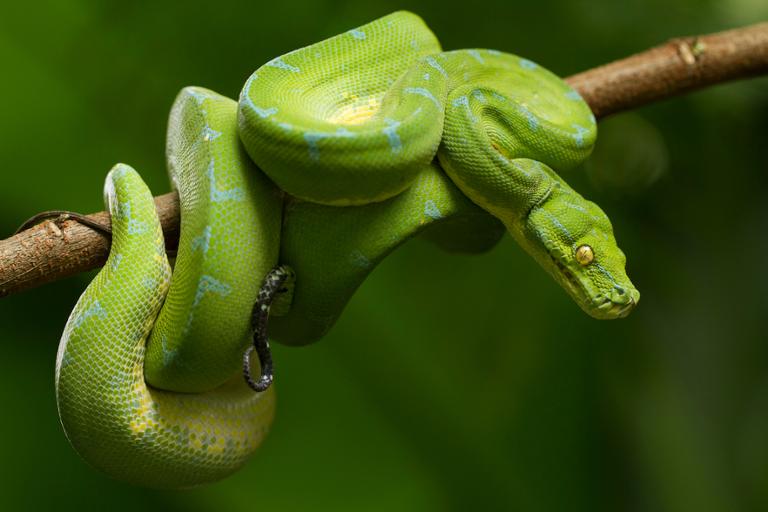
As a novice Python developer, the world is your oyster with regards to the type of applications that you can create. Despite its age (30 years—an eternity in tech-world terms), Python remains a dominant programming language, with companies using it for all kinds of services, platforms, and applications.
For example, Python lets you create web applications via Django or other frameworks such as Flask. Perhaps you want to create games instead? For that, learn Pygame for 2D games (or Panda3D for 3D). Or maybe you’re more enterprise-minded, and want to create useful utilities (such as automatically cataloguing e-books); in that case, Python works well with frameworks and software such as Calibre.
Thanks to Python’s popularity, it’s also finding its way into niche and nascent industries such as machine learning and artificial intelligence (A.I.), as well as specialized industries such as finance. In other words, Python isn’t just good for building things today; its utility is likely to last far into the future.
Getting Started
Before you can start writing applications, there's a few things you need to learn about Python and how it interacts with packages and modules; we’ll cover these through the rest of this short introduction. (Emphasis on “short”; we’re going to focus on what’s important.)
Packages and Modules
Python lets you bundle up functions, classes and variables into modules as a way of managing complexity. In Python, modules are the equivalent of libraries or standard libraries in other programming languages.
You can wrap a Python application’s module into a hierarchical file directory structure that consists of modules and sub-packages and sub-sub-packages. Creating packages is a more advanced feature (not something to learn as a novice), but using modules and packages is something everyone interested in Python should know. Note, Python has built-in modules but not built-in packages; you have to install those.
Here's a full list of built-in modules. There are approximately 250 of them as of Python 3.8.1. This is an example of what you need to import the shuffle function from the built-in random module:
from random import shuffle
You can also import everything from modules (check out this example below, which involves importing from random and math). The example prints the value of pi from the math module and generates a random int between 0 and 99:
import random
import math
print(math.pi)
print(random.randrange(100))
Python has an incredible number of packages available to install, all free; those contain modules and individual Python files. Currently there are 214,730 packages in the Python Packages Index PyPi.
The usual way to install packages is with a utility called pip. Installing packages can be a little messy but we're stuck with it for the time being. If you do it wrong, you can end up with packages that can only be installed or removed using root. You will need to get familiar with the term "virtual environment."
What is a Virtual Environment
It's a way of installing packages for an application rather than your Python interpreter. This is because packages are versioned, and you may need different versions of the same package in different Python applications. The idea is that you can set up as many virtual environments as you want, but only one can be your currently active environment. Any package you install goes into the currently activated virtual environment.
In Python 3.x, you can use the venv
command to create virtual environments. But it’s not the only way, as we’ll see with conda below.
Let’s look at one of the most popular packages: numpy. If you want to use numeric arrays in Python, do not use the built-in arrays module, which is slow; instead, install numpy, which has been programmed (In C) to do very fast array handling.
Conda, miniconda or Anaconda?
Having said that you install packages with pip, the messiness arises because some packages have their own packaging. NumPy can be installed with pip, but most often you'll use conda, which is an environment manager and a package manager. Confusingly, you have to first create a virtual environment and install conda into it using either miniconda or the full anaconda installers.
Anaconda includes 150 scientific packages (and gives you access to over 1,500 in total). It is probably easiest to get started with, so long as you don't mind 3Gb of disk space being eaten up! If you go for miniconda, you still have to manually install the packages afterwards with either pip or conda (more confusion!). Pip installs Python packages within any environment; conda installs any package within conda environments.
Conclusion
Virtually every Python program will need to import code from one or more modules, so you need to understand them, and you will need to install code from packages, as well. Make sure you are also familiar with virtual environments, and use packages with their own environment managers (like conda) to do the heavy lifting. Python is popular, so the more you know about it, the more opportunities you can seize upon!