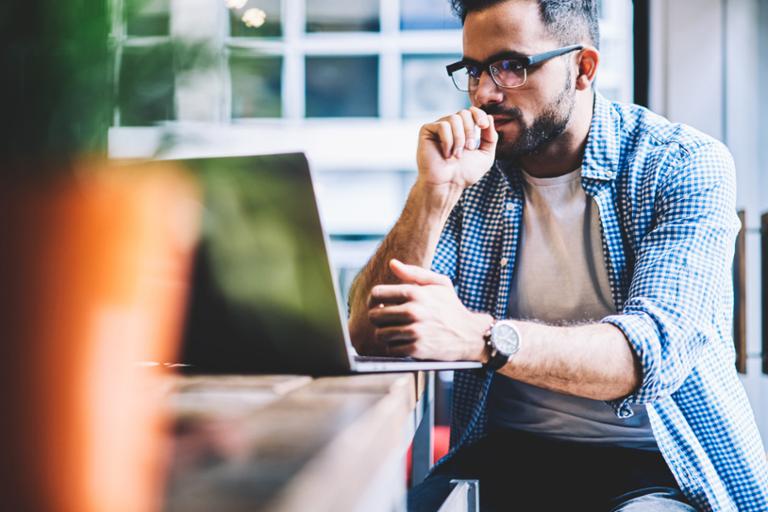
Since its first appearance in 2002, the .NET framework has been a major success for Windows. Along the way, it spawned the .NET Compact Framework on Windows CE, as well as the .NET Micro Framework (targeted at embedded devices with limited resources). Mono, a version of the Common Language Runtime (the Virtual Machine that runs .NET code), and its C# compiler were developed for Linux and Mac operating systems. Some devs feared that Microsoft would use its patents to squish Mono (which is part of the non-profit .NET Foundation), but those suspicions have proven groundless (so far, at least). Microsoft bought Xamarin, the company that developed Mono, and incorporated it into the .NET Core. Indeed, the past seventeen years have seen the rise of many open-source .NET projects such as ASP.NET MVC, ASP.NET Core, Entity Framework, MSBuild, NuGet, .NET Core and others. In other words, this is a healthy ecosystem to explore. Let’s take a (brief) look at .NET Core, including its speed on various platforms. The .NET Core is particularly worth exploring, given its cross-platform abilities when developing apps for a number of devices and form-factors.
.NET Standard
Because there are so many different combinations of .NET APIs (including .NET Framework and .NET Core), Microsoft has devised a standard called .NET Standard. This helps in building applications for different versions of .NET. (There's nothing worse than trying to move an application built on .NET Framework to .NET Core on Linux and discovering you've used a feature that's not available on the other platform—if you get that issue, try running it under Mono, which supports many features up to .NET Framework 4.7.2.) The table on the .NET Standard web page lists the versions of .NET Standard (from 1.0 to 2.0), and shows which framework versions are compatible. If you want to write an application that can target .NET Standard 1.6, this equates to .NET Core 1.0, .NET Framework 4.61, Xamarin.iOS 10.0, etc. Before you start with .NET Core, you should definitely read the online documents first. Currently, the highest stable version of .NET Core is 2.2, but with the launch of Visual Studio 2019 at the start of April, the crown was taken by .NET Core 3.0, which includes C# 8.0, WPF and WinForms, and a lot more. For the purposes of this article, I used .NET Core 2.2. You can download it for your platform of choice (Windows, Ubuntu, Red Hat, etc.); just start here. I'd recently updated my Ubuntu to 18.04LTS and installed onto that. However, if you are on Windows, you can also install .NET Core side-by-side with .NET Framework. Depending on your flavor of Linux, you will have to add export commands so the path to dotnet works from any folder. The instructions explain what to do (I added two lines to the .bashrc in my home folder). After the install, a quick dotnet -hin a new terminal will confirm everything is working.Some Complications
Curious, I installed Visual Studio 2019 Community Edition on Windows; this is the preview version that supports .NET Core 3.0. I created a project in order to run it on my Windows PC, but I wasn't initially successful with that, so switched to 2.2. To compare speeds (Windows 10 vs. Ubuntu), I created a program using the algorithm given in the first answer here. It calculates Pi to however many digits you specify; I went with 10,000 digits. I've snipped out the function, but just copy it from the first answer on that Stack 0verflow link:using System.Diagnostics; using static System.Console; public static string CalculatePi(int digits) {... snipped } static void Main(string[] args) { var stw = new Stopwatch(); stw.Start(); var piStr = CalculatePi(10000); stw.Stop(); WriteLine($"It took {stw.Elapsed} to calculate pi to 10000 digits"); WriteLine(piStr); }. I copied the solution folder over to Ubuntu and then compiled it from a terminal. For Windows 10 (running in release mode), it took 5.11 seconds to run. On Ubuntu, it took 13.67 seconds. I tried it with .NET Core 3.0 on Ubuntu, compiling the solution from the command line, but received an error. It compiled once I edited the .csproj and changed it from version 3.0 to 2.2, then ran the compile with sudo to elevate it.
sudo dotnet build ex3.sln Microsoft (R) Build Engine version 15.9.20+g88f5fadfbe for .NET Core Copyright (C) Microsoft Corporation. All rights reserved. Restore completed in 78.9 ms for /home/david/sharedfolder/Code/ex3/ex3/ex3.csproj. ex3 -> /home/david/sharedfolder/Code/ex3/ex3/bin/Debug/netcoreapp2.2/ex3.dll Build succeeded. 0 Warning(s) 0 Error(s) Time Elapsed 00:00:02.93. (By coincidence, I recently read an article that suggested running code in a VM such as VirtualBox or Hyper-V can incur a greater processing penalty. This is definitely worthy of further investigation.)