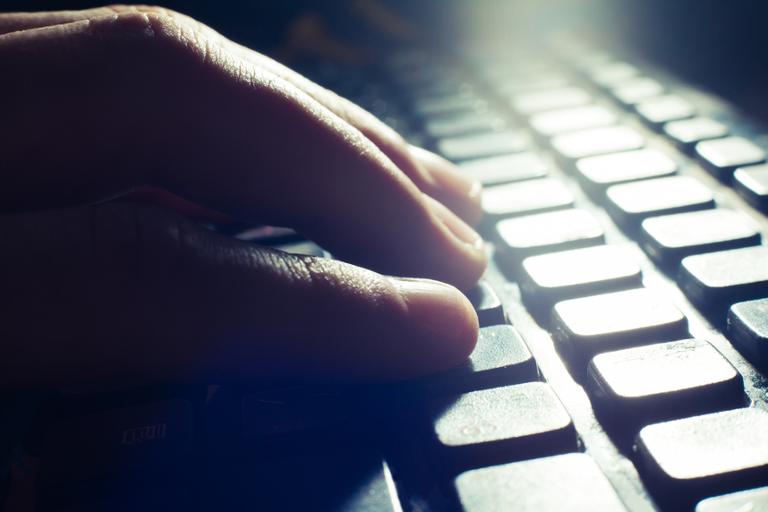
PHP is the traditional server language for developing Websites. It’s free and ubiquitous, undergirding hundreds of open-source applications such as WordPress and Drupal. Getting started is very easy, and hosting costs can be very cheap for LAMP technologies (including Linux, Apache, MySQL and PHP). Despite its ubiquity, development in PHP can often prove frustrating; the language feels like a hodgepodge with (for example) no single name standard for functions. Any major development in PHP requires using a framework. In the past, I created small and medium Websites in PHP and managed to make them reasonably secure. These days, though, the list of potential vulnerabilities would make me hesitate to start from scratch. Instead, I’d choose a framework and a different programming language. One development language that I'd consider for Websites would be C# in the ASP.NET MVC technology, but since it seems to change every year or two (making it hard to keep abreast of latest developments, so to speak), I've been looking at Python. Compared to PHP, the Python language has been carefully developed, with new features road-mapped well in advance of releases. The syntax is cleaner than PHP (no braces!) and finding out what's gone wrong in the code is a lot simpler. For Python, it also makes sense to use a Web framework, unless you want to code a URL router, a templating system, and manage database connectivity. The very smallest website in Python is this:
def application(environ, start_response): start_response('200 OK', [('Content-Type', 'text/plain')]) yield 'Hello World\n'But first things first: you need to set up Python to work with Web applications. There are a variety of ways to do this. Remember that it's not just about being able to write Python programs and view them in your browser, but also moving them into a production environment. To that end, I played around with several frameworks, including the micro-framework flask, but had a little bit of trouble getting it working. One problem I came across repeatedly involved a syntax error with the line:
from flask import FlaskThat's a common line with the flask micro framework. Here’s the problem: while I was using a default Python (2.7.6), some of the linked modules were 3.4.3; since the pip installer defaults to version 2.7, the flask package was missing. The answer is to install pip3, just like StackOverflow suggests. Problems like this can waste a lot of time. Who said Python development was frictionless?
Web2py
Another viable Python framework is web2Py. Installing it on Ubuntu is easy: just unzip the example files into a folder, then do python web2py.py; type in a password for the administrative interface and it defaults to localhost port 8000. There are also installers for Windows and Mac. I installed it on Ubuntu running under VirtualBox with these seven commands:sudo apt-get update sudo apt-get install unzip cd ~ wget http://www.web2py.com/examples/static/web2py_src.zip unzip web2py_src.zip cd web2py python web2py.pyAfter installing web2Py, you can use it to create a Web application—but there is a learning curve. For those who like meaty documentation, Massimo Di Pierro the author of web2py has provided a Creative Commons-licensed reference manual in PDF format. Web2Py is MVC (Model-View-Controller)-based, with an interface that consists of two models (db.py and menu.py), two controllers and 15 views. Static pages, known as flatpages, are provided for 403, 404 and 500 errors, as well as css, fonts, images and javascript files. This link goes to the layouts page, with nearly 100 (free) downloadable plugins. Web2py uses Python for its models, controllers, and views, though Python syntax is modified in the views to allow more readable code. Python code is embedded in HTML inside double braces {{like this}}. Indentation is done for html, not Python, and web2py allows for “unindented” Python. As indentation is normally a key part of Python, this is overcome by using the word pass. So this in Python:
if i == 0: response.write('i is 0') else: response.write('i is not 0')Becomes this in web2py:
{{ if i == 0: response.write('i is 0') else: response.write('i is not 0') pass }}Along with the administrative interface, there is an Examples app and a Welcome application that's the basic template for any web2py application, and is known as the scaffolding application. When you create an application, this scaffolding application is cloned, and you can start by customising it, defining the data in the models, controllers and views.